Data Structures and Algorithms in Java
by EDUCBA

This lesson is E-learning lesson.
About the Class
- Class Level: Intermediate
- Age Requirement: 15 to 99 years
What You'll Learn
Course Objectives
At the end of this course, you will be able to:
- Know the basics of Object Oriented Programming
- Use all the syntax of Java programming language with ease
- Know about the data structures and algorithms used in computer programming
- Understand the way in which data is stored or arranged in your computer memory
- Know more techniques in data structures and algorithms
Prerequisites for taking this course
You need to have a basic knowledge in programming. You need to know the basics of computer. If you wanted to run the example programs then you need to have MS-DOS in Microsoft windows or a similar text based environment.
Target audience for this course
IT professionals who are dealing with Java programming can take up this course. Computer science students and anyone who is interested in learning about the Data structures and Algorithms in Java can also take up this course.
Course Description
- Section 1: Introduction to Data Structure
Course Structure for DSA
This section gives a brief introduction to the Java programming language, Data structures, and algorithms in Java. The section also includes the definition for few of the frequently used terms in this course like database, record, field, key and others.
More Details on DSA
This chapter tells you what are the information which will be covered in this course about data structures and algorithms in Java. It gives a chapter wise brief introduction. - Section 2: Classes and Objects
Java Objects and Classes
In this chapter, we will see what are objects and classes in Java. The object includes Methods and Variables. The other topics included in this section are
Creating and using objects
The Dot Operator
Defining a Class
Modifiers – Access control, Static, Abstract, Final
Declaring Instance variables
Declaring Methods
Different Types of Code Constructor
Constructors are used to initializing a newly created class. The section also explains the different types of constructors like a class constructor, Code constructor and argument constructor with examples of Java programs for easy understanding.
Java Keywords and Java Comments
This section gives a list of keywords in the Java Programming language. There are around 50 keywords in Java and each is explained with a brief description and an example.
Basic Java Commands are also included in this section explained using a simple Java program.
Sources File Declaration Rules
In this chapter, you will learn how to write a source file in Java, the behavior of Java compiler and JVM in running and compilation.
Java Basic Data Types
Basically, there are two data types in Java – Primitive and Reference/Object Data Type. Primitive data types are divided into eight and include Byte, short, int, long, float, double, boolean and char. Each data type is given in detail with an example. - Section 3: Decision Making
Decision Making
Decision-making structure in Java is where a condition is to be tested by the program and if the result of the condition is true then the particular set of statements are to be executed and if the condition fails then the other set of statements are to be executed. The decision-making structure is explained with a simple program.
The different decision-making statements are if statement, If else statement, nested statement and switch statement. All these statements are given in detail in this chapter.
Switch Case Program
The switch statement lets a variable to be tested for equality against a set of values. An example program is given for easy reference.
Java Loops and Control System
Loop statement in Java helps to execute a statement a multiple numbers of times in the programming. There is a different type of loops available in Java – while loop, for loop and do while loop. This section contains an explanation for these loops.
Loop control statements change the execution method from its normal way. Break statement and continue statement are different control structures. - Section 4: Numbers Class
Java Number
Numbers use primitive data type often. These are called wrapper classes which come under the number. There are a lot of instance methods that are used under number class. These methods are mentioned with a brief description in this chapter. Few methods are compareTo(), equals(), valueof() and others.
Java Lang Math
Java Lang Math is again a wrapper class which is explained in this section - Section 5: Character class
Java Character Class
This chapter contains the following topics under it
What is a character class
Escape sequences and example
Character Methods and example
Java Lang Character – Eclipse - Section 6: String Class
Java String
Strings are a sequence of characters in Java. Java offers the string class to create and manipulate strings. The major topics included under this section are
Creating strings
String Length
Concatenating strings
Creating format strings
String methods - Section 7: Arrays
String Fundamentals
This section again explains the fundamentals of using a string in Java programming language.
Array to for Each Iteration
In this chapter, you will learn how to use the for-each loop in Java with arrays. - Section 8: Advanced Arrays
Java Array
An array is a storage place which stores a sequential collection of elements which are of the same type. This tutorial contains the following subheadings
Declaring Array variables
Creating arrays
Processing arrays with example
The for each loop
Passing arrays to methods
Returning an array from a method
The arrays class
Java Date and Time
The date class in Java is available in java.util package and has two constructors. The different methods of data class are also explained in detail in this section. The other topics included are
Getting current date and time
Date comparison
Date formatting
Date format codes
Date and Time conversion characters
Parsing strings into dates
Measuring elapsed time
Sleeping for a while
Gregorian calendar class - Section 9: Regular Expressions
Java Regular Expression
Regular expression helps to find out matches of strings or set of strings using a special syntax. It can also be used to edit or manipulate the data. There are three main classes – Pattern class, Matcher class, and PatternSyntax Exception.
Long String and String stream
This section helps you to understand how to convert String into an InputStream in Java.
Or Clause Situation
This section explains how the AND or OR in IF statement is used in Java. Examples are given for reference.
Example of Pattern Matching
Java has a package for pattern matching under regular expressions. This is explained under this section with few examples for easy understanding.
Study Methods
Study method reviews the string and returns a boolean value saying that the pattern is correct or not. This concept is explained in this chapter.
Append Replacement and Tail Method
The matcher class provides an appendReplacement and appendTail method which is used for text replacement. These two methods are given with examples in this tutorial.
Java Methods
Java methods are a collection of statements used to perform an operation. The other topics included under this chapter are
Creating methods with example program
Method calling
The Void Keyword
Passing Parameters By Value
Method overloading
Using Command line arguments
The this keyword
The constructors
Variable arguments
Finalize method - Section 10: Methods and File IO
Different Types of Methods in Eclipse
This chapter explains what is an eclipse in java and the different perspectives of the eclipse. It also lets you learn how to install, configure and run eclipse in Java
Java Files Input and Output
Streams represent a sequence of data. The two kinds of streams InputStream and OutputStream are explained in detail under this section. This chapter also includes the following subheadings in detail with examples wherever needed.
Byte streams
Character streams
Standard streams
Reading and writing files
File InputStream
File OutputStream
File navigation and I/O
Creating and listing directories in Java
Java Exceptions
An exception arises during the execution of a problem in Java. The exception occurs because of various reasons. Three types of exceptions are Checked Exceptions, Unchecked Exceptions, and Errors. The exception hierarchy and exception methods are also explained in detail in this chapter. - Section 11: OOPS Concepts
Object Oriented Concept
In this section, we will learn about basics of OOPS, the concepts of OOPs in Java and advantages of OOPs.
Using The Super Keyword
The super keyword is a reference variable in Java which is used to refer parent class object. It includes the usage of Java super keyword
Virtual Methods
Virtual function or virtual method is an overridable function in Java. It is an important part of OOPs. The concept of virtual function is explained in detail under this chapter with examples.
Java Inheritance
Inheritance means the process through which the properties of one class is acquired by another. The types of inheritance are demonstrated in this chapter.
Rules for Methods Overriding
This section explains what is overriding in Java, benefits of overriding, rules for method overriding and using the super keyword with an example.
Introduction Java Abstraction
Here you will learn what is an abstraction, abstract classes, inheriting the abstract class and abstract methods with an example.
Java Encapsulation
Encapsulation is one among the four concepts of OOP. This section explains the meaning of encapsulation in Java and the benefits of encapsulation.
Java Interfaces
The interface is a reference type in Java and is a collection of abstract methods. The other topics included in this section are
Declaring interfaces
Implementing Interfaces
Extending interfaces
Extending Multiple Interfaces
Tagging Interfaces
Java Packages
Package means a grouping of related terms. The chapter includes the following topics
Creating a package
Import Keyword
Directory structure of packages
Collections In Java
Under this chapter, the collection framework is explained along with its goals. The several interfaces of the collection framework are also included under this chapter. - Section 12: Multi-Threading Overview
Contents of Multi-Threading Overview
A multi-thread program can handle a variety of tasks at the same time. The other topics included are
Life Cycle of a thread
Create Thread by Implementing Runnable Interface
Create Thread by Extending Thread Class
Methods of Threads
Java Multithreading concepts
Concurrent Access of Data - Section 13: Concurrency
Java Concurrency Multi-Threading
Multithreading means there will be multiple threads of execution inside a single program. It is a great way to increase the performance of the program. An example of Java Concurrency Multi-Threading is also included in this chapter. - Section 14: Types of Concurrency
Multi-Threading Benefits
This chapter includes the benefits of multithreading in Java.
Concurrency Models
In this chapter we will see what is concurrency model, parallel workers of concurrency model, types of concurrency models, assembly line, Job ordering is possible and the different types of concurrency models.
FAQ’s General Questions
- Who can take the Java training?
This course is open for working professionals, current graduates of computer science field and also to anyone who is interested in learning this language. You don’t necessarily need to have a prior programming experience - What will I be able to do after completing this course?
You will be able to write and test Java programs on your own. You will also be able to solve computational problems in Java in a new approach. It will improve your career and job performance.
Fee Includes
Lifetime Access to eLearning content
Why Book Through LessonsGoWhere?
- Booking is safe. When you book with us, your details are protected by a secure connection.
- Secure your slot instantly. Book classes with us and your seat is confirmed immediately.
- Earn Reward Points. This class will earn you upto 140 reward points. Points can be used for a discount off your next class!
Questions about this class?
Get help from knowledgeable expert.
Together with eduCBA, we bring you an amazing course on Data Structures and Algorithms in Java.
In-depth Conceptual Analysis and Programming hands on in Data Structure and Algorithm in Java.
Java Language
Java is a modern object-oriented programming language. The Java language is more easy language when compared to C and C++. A lot of websites and applications are running using Java as it is fast, secure and reliable. Java is free to download
What are Data Structures and Algorithms in Java
Data structure means an arrangement of data in a system’s memory. The characteristic of the data structure includes Array, Ordered Array, Stack, Queue, Linked list, Binary tree, Red Black tree, 2-3-4 tree, Hashtable, Heap, and Graph. Each of these characteristics of data structures has its own advantages and disadvantages.
Algorithm means manipulating the data in these structures in different ways. The important algorithm category is sorting the data. There are several ways to sort the data. Recursion is an important concept in designing certain algorithm.
All e-learning lessons bought through LGW will be final and no refund, return, cancellation or exchange will be allowed.
Frequently Asked Questions 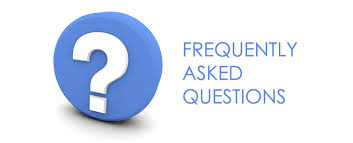
Have a question about LessonsGoWhere? We've collected all your questions and our answers into a convenient list here. If you have any questions, please don't hesitate to email us at info@lessonsgowhere.com.sg
Q: What's LessonsGoWhere?
A: LessonsGoWhere.com.sg (LGW) is Singapore's first online marketplace to list, discover and book in-person courses. You can shop, compare and review lessons on LGW, across areas like Baking, Cooking, Music, Fitness, Yoga and even Exotic lessons!
Q: Are the classes I find on LessonsGoWhere online lessons or are they conducted in real life?
A: All the classes you can find on LessonsGoWhere are lessons that are conducted in real life, by real people. We sincerely believe in the importance of the human touch and that we can build bonds and relationships through shared passions. Would you like to learn SCUBA diving through an online tutorial? We didn't think so.
Q: Who are the teachers in the classes available on LessonsGoWhere?
A: The classes on LessonsGoWhere are taught by professional trainers, instructors, chefs and coaches, as well as passionate individuals who want to share their experience and knowledge. LessonsGoWhere does not restrict lessons from freelancers or other qualified individuals. However, we are very strict on the quality of lessons and if we receive complaints regarding the quality of the lessons from our users, we will not hesitate to take action in removing the lessons and banning the lesson provider.
Q: What types of lessons are offered on LessonsGoWhere?
A: There are a wide range of lessons on various topics and areas of interest on LGW. The main categories right now are Baking, Cooking, Music, Sports, Art, Yoga and Exotic lessons. However, we are always looking out for more lessons to add to the marketplace. If there's a particular category of lessons you'd like to see, please don't hesitate to let us know at info@lessonsgowhere.com.sg
Q: Are the lessons real? Will I get scammed if I book classes on LGW?
A: The lessons are definitely real. All lessons are uploaded and checked by a team of hardworking elves (the founders of LGW) who work tirelessly and through late nights to ensure that the details are accurate. All lesson providers are also contracted with LGW to provide the lessons. We back our lessons up with a 100% Refund Policy. In the scenario that a lesson is cancelled, we GUARANTEE that we will refund you 100% of the fess paid. The security of our customers is our number 1 priority. If you have any queries on the lessons or the security of the website, do not hesitate to email us at info@lessonsgowhere.com.sg
Q: Why do I need to pay immediately?
A: We require that you pay for the lesson in full before you are issued an email confirmation of your booking for the lessons. There will be costs incurred by the instructor before the lesson commences, and your payment not only immediately confirms your booking, but will enable us to pay the lesson provider immediately!
Q: Why should I book and pay for my lessons on LessonsGoWhere.com.sg?
A: In cases of disagreement between you and the lesson provider, LGW will have a copy of your booking details logged with us and can also withhold payment from the lesson provider. Your booking details will be helpful should any disputes arise in terms of bookings and payments. Also, with the wide variety of lessons on LGW, you can immediate compare and choose your choice of classes at your convenience! You can also contribute to the community by reviewing the classes and lessons you've attended, earning you Reward Points, and also helping potential students make better choices and their reviews will benefit you too!
Q: What happens after I've made payment?
A: Once you've confirmed payment for the lessons of your choice, you will receive an email confirmation from us, letting you know the date, time and location of the lesson. On the day itself, simply present the email confirmation to the lesson provider and you will be able to attend the lesson!
Q: What happens if I cannot attend the lesson?
A: In the case that after you have booked your lesson, but are unable to attend, let us know immediately. Email us at info@lessonsgowhere.com.sg. We will try our best to transfer your booking to another time, or if you prefer, to a friend. While we cannot refund your payment if you are unable to attend, let us know and we will try out best to accommodate you!
Q: What if the lesson provider asks for more money when I arrive?
A: The pricing information for each lesson is clearly stated on each listing and will also note what is or is not included. If you encounter a lesson provider who asks for more money on top of the payment you have already made to us, please contact us immediately at info@lessonsgowhere.com.sg and we will try our best to rectify the situation.
Q: Do the fees include equipment and location rentals (if necessary)?
A: While some lesson providers will include equipment and facility bookings with the fee, others might not. Don't worry though, the pricing information is clearly stated on each listing and will also note what is or is not included. If you are still in doubt after checking the listing, you can email us at info@lessonsgowhere.com.sg and we will clarify the issue for you.
Q: What happens if I pay for a lesson and the lesson provider cancels or doesn't respond?
A: Don't worry! If the lesson is cancelled or if you are unable to get a response from the lesson provider, email us at info@lessonsgowhere.com.sg and we will refund you 100% of the fees you paid.
Q: My friend/girlfriend/boyfriend/family member wishes to attend the lesson as well, can I book for them too?
A: Yes! Learning is always an experience best shared. It's also a great activity to bond over! If you have others who are interested in attending the lesson as well, simply book the appropriate number of slots for the lesson and they can accompany you. Book fast though! Most lessons only have a limited number of slots available and if you aren't fast enough, you might not be able to secure the slots for them!
Q: Should I leave a review after I've attended the lesson?
A: Definitely! Not only do other students benefit from your review of the lesson, you will also receive Reward Points for your review! You can use those Reward Points as a discount off your future lessons too! Everyone benefits!
Q: Are the reviews posted on LGW true?
A: Each review posted on LGW will be monitored by our administration team. We try our best to create a helpful and engaging community and we do not like foul language, sexual themes, trolls or spammers. But yes, all reviews are unedited by us and are the opinions of the reviewer.
Q: Are you Baking and Cooking courses Halal certified?
A: Halal certification is a type of certification given only to restaurants. Most of our classes use pork free ingredients. For more information, please get in touch with us to find out more!
Found the answer to your questions? Book your lesson now!
Ready to take this class?
Reviews of Classes by EDUCBA
Heow P.
15 Mar, 2021
Andy K.
8 Jun, 2020
poor in presentation & speech
Wei Tian Edwin Y.
4 Jun, 2020
Wei Tian Edwin Y.
4 Jun, 2020
Anand K.
12 Apr, 2017